Abstract
The lessons from the previous chapters are here applied to a very brief discussion of numerical techniques. First we show how to calculate the capacitance of three-dimensional structures; we then follow this with similar calculations of inductance. Both of these are well defined in the long wavelength approximation. We then describe how a full wave numerical solutions can be implemented using the popular method-of-moments. We follow this by discussing how to implement excitations or wave ports and how to implement boundary conditions between different dielectric layers.
6.1 Introduction
This chapter will take our experience from Chapters 4 and 5 and use some of these results to sketch how simulators work. We will not go into great detail, as it is somewhat outside the scope of the book, but from what we have learned so far we can draw some interesting conclusions about what will make a good simulator and how a poor one may be revealed. In fact, this chapter will show yet another application of estimation analysis, although the context is quite different. We will make simplifying assumptions and briefly discuss the solution and verify/evaluate the result. There are many details when implementing a real-world simulator that fall outside the scope of this book, and we provide the interested reader with avenues to explore further in the References section below.
We start with the simple case of electrostatics where we show how charge induced from a voltage can be solved by, in principle, quite simple methods. After that we investigate inductance simulations by calculating fields from current distributions. We proceed by showing some of the basic steps necessary to design a full-wave simulator, and what an excitation port is and how it is used. Finally, a brief overview of Matrix inversions is provided.
6.2 Basic Simulator Principles
All simulators follow the same basic principles: divide the subject matter (be it time or space) into smaller chunks, referred to as a grid or mesh. Assume that inside each chunk, the property of interest is not varying at all or is varying slowly, either a constant or some linear and perhaps some higher-order polynomial. Set up the governing equations with these approximations in mind and solve them (nearly always by inverting a matrix). Repeat by refining the grid until the desired accuracy has been reached. These same principles can be found in circuit simulators, Maxwell field solvers, system simulators, etc.
For our purpose, we would like to see how field solvers operate. We have seen in previous chapters that the field from current segments can be solved precisely and, as the reader has probably guessed by now, some field solvers work by dividing up the current-carrying conductors into smaller chunks where each chunk has a constant current. Similarly, if we want to know capacitance, we divide the surface of each conductor into smaller pieces where we assume voltage and charge is either constant or slowly varying in some cases.
6.3 Long Wavelength Simulators
Long wavelength simply means that the length scales of the problem are much smaller than any field wavelength, as we saw in Chapter 4. The vector potential, AA, will give rise to magnetic fields and thus inductance, whereas φφ will create electric field and capacitance. We will take a look at these effects in turn.
Capacitance Simulations in Three Dimensions
We know from Chapter 4 that the long-wavelength approximation means the length scales of the problem are much smaller than any wavelength of the fields. For the potential field, we have from (4.29) that
outside any conductor (where the charge is zero). This is simply Laplace’s equation in three dimensions. It may be tempting to attempt to solve this equation with a given set of boundary conditions. It certainly looks fairly innocuous, but the main difficulty one runs into is one of accuracy. The result of the simulation will be induced charge on the surfaces of various conductors. To calculate the charge will involve taking the second-order derivative of φφ at the surface. We know from the equation itself this derivative is zero outside. In order to implement something like this, the grid needs to be really fine and it will take a long time to solve. Instead, it is more efficient to start from (4.37) to calculate the induced charge. Here, we divide the conductors into smaller shapes where we assume the charge is constant, and using (4.37) we then calculate the potential this charge distribution produces at a certain point xx. We then simply go through all the conductors, calculate the voltage resulting from everything else, and end up with a matrix equation.
We will illustrate this process by making some simplifications to illustrate the main point. It is a good example of estimation analysis but the context is a little different from the rest of the book. The estimation analysis will here result in a set of pseudocodes that could be implemented. The reader must bear in mind that following these procedures will produce codes that work in principle. Building codes that are efficient and void of bugs is a whole different cup of tea and way outside the scope of the book.
Simplify
We now make a number of simplifications:
The conductors are perfect, so no charge will penetrate into the volume of the conductors, i.e., they are surface charges.
We divide each conductor surface into much smaller surface segments where the charge is constant: see Figure 6.1.
There is only one dielectric medium, so no dielectric boundaries need to be considered.
We will ignore the complication of self-interaction where a given charge impacts the voltage on its own segment (there is a singularity). This is an important effect and not that difficult to deal with, but for illustrative purposes we will not address this problem here.
Figure 6.1 Conductors in three dimensions where the surface is divided up into smaller areas within which the charge is constant.
Solve
Let us now define the problem by looking at the potential from a charge on a surface segment jj, at a point rr. We have from equation (4.37)
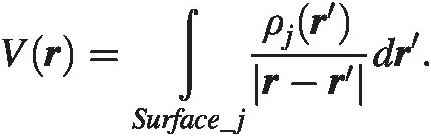
We now calculate this voltage at a point in the center, riri, of another segment ii and sum over all segments. We find

This can be written as a matrix equation:
From the boundary condition we know the value of VV on all surfaces, and we can calculate GG and solve for ρρ. To get to capacitance, let us be a little more careful with notation. Let us assume we have NsegNseg conductor segments, each of which is subdivided into NsubNsub charge segments. We have a total number, Ntot = NsegNsubNtot=NsegNsub of charge segments. Let us denote by ρijρij charge segment jj on conductor segment ii. In order to find the capacitance between conductor segment ii and jj, we need to ground all segments except ii, which has a voltage of Vi = VVi=V. We solve (6.1) and calculate the capacitance from (4.48)

With this procedure one needs to go through all conductors and set each voltage to VV one at a time, keeping all others grounded, solve the equation, and calculate the resulting capacitance. It sounds easy enough and it sort of is, but the difficulty lies in the details. The integrand has a divergence when i = ji=j that is fairly strong and the accuracy required in the numerical integration can be excessive. Furthermore, the grid is critical and in order to set it up properly one has to have a deep understanding of how charge will distribute itself on the surfaces beforehand. However, the pseudocode is straightforward.
Pseudocode
Subroutine build_G(Mesh)
* Loop over Mesh to build Matrix G.
For i = 0,N do
For j = 0,N do
Integrate_element(r[i],r[j])
End for
End for
End build_GSubroutine CapacitanceSolver
Create_mesh(Mesh)
G = Build_G(Mesh)
Ginv = Invert_matrix(G)
For i = 1,N do
V = Define_voltages(Mesh)
rho = Multiply(Ginv,V)
C[i] = sum(rho) // Assuming the voltage V = 1.
End for
End CapacitanceSolver
Verify
Simulators are traditionally verified by having them solve known problems and comparing the solutions. We leave it as an exercise to the reader to implement a code and gain experience by learning by actually doing.
Evaluate
We are not going to go to the trouble of actually building codes here, but simply state that although this may sound really simple and in some meaning of the word it is, the problem comes when you want accuracy. The charge tends to want to sit at the edges of the conductors, similar to the current distributions we examined in Chapter 5. This means that around those edges the surface elements must become really small to resolve the charge distribution, adding up to quite a few elements for large geometries. Some serious tricks need to be employed to get to the bottom of this and make the problem manageable. The most successful simulators have in common that they have found clever ways to manage the grid-generating algorithms. This has made some inventors very wealthy indeed. The construction of the grid and the speed with which the matric, GG, is calculated are critical parts of building simulators. If this is done poorly it will take a long time to find a solution for a given accuracy.
Inductance Simulations in Three Dimensions
Inductance simulators can be made in a similar fashion, where we divide up the conductor into smaller current-carrying segments. The steps we need to go through are somewhat more complicated, and we will just sketch the procedure here. Far more details can be found in [3].
Simplify
We assume we are dealing with one long wire of a certain thickness and width that are much smaller than the conductor length. We chop the wire into small conductor segments where we assume several things:
The magnitude of the current in each conductor segment is the same, so there is no capacitive or other loss.
The current is uniform in a cross-section of the wire, in other words skin depth is not important. Each conductor segment can then cover the full cross-section of the wire.
These is only one magnetic medium, so no boundaries need to be considered.
We will ignore the complication of self-interaction where a given current segment impacts its own magnetic field (there is a singularity). This is an important effect and not that difficult to deal with, but for illustrative purposes we do not address this problem here.
Solve
Let us now define the problem by looking at the vector potential from a current on a conductor segment ii, at a point rr. We have from (4.36)

With this expression we can now calculate the partial inductance matrix, Li, jLi,j, between segments i, ji,j, using (4.46) and
We find

We refer to the case i ≠ ji≠j as the mutual inductance and i = ji=j as the self-inductance, similar to what we discussed earlier. Here we see explicitly the convenient fact that when the two currents are perpendicular to each other their mutual inductance is zero. If we decide not to divide the conductors – perhaps they are really thin, or frequency is low – we will be done here. We merely have to sum up all the elements to get the total inductance of the wire.
